Submitting API Requests with Python 3 - A Tutorial
In this tutorial, we will create an API request with Python 3 using the Requests package. Requests is an elegant and simple HTTP library for Python that you can install using pip.
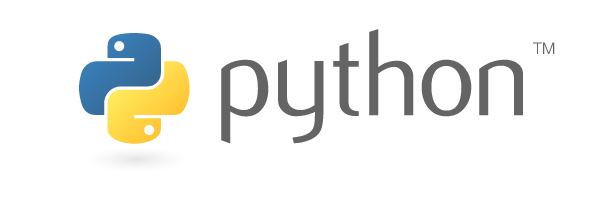
In this tutorial, we will create an API request with Python 3 using the Requests package. Requests is an elegant and simple HTTP library for Python that you can install using pip.
Installing the Requests Package
To install Requests globally, use the following command:
$ pip3 install requests
If you do not have Python 3, please see my Python 3 installation guide for instructions on how to install Python 3.
Postman Echo
In this tutorial, we will use Postman Echo to test our API calls with Python 3.
Postman Echo is a service you can use to test your REST clients and make sample API calls. It provides endpoints for GET
, POST
, PUT
, various auth mechanisms and other utility endpoints.
The documentation for the endpoints as well as example responses can be found at https://postman-echo.com.
The API Endpoint we are going to use is https://postman-echo.com/get?foo1=bar1&foo2=bar2
Python GET
API Example
# Here we import the request library
import requests
# Here we define the api-endpoint
url = "https://postman-echo.com/get?foo1=bar1&foo2=bar2"
# BASIC Authentication
API_USERNAME = 'postman'
API_PASSWORD = 'password'
# Here we define the headers
headers = {'content-type' : 'application/vnd.json+api'}
# Sending GET request and saving response as response object
r = requests.get(url, headers=headers, auth=(API_USERNAME, API_PASSWORD))
# Printing the response object
response = r.text
print("Response:%s"%response)
In your terminal you want to run:
python3 [file_path]
You should see the following output:
Response:{"args":{"foo1":"bar1","foo2":"bar2"},"headers":{"x-forwarded-proto":"https","x-forwarded-port":"443","host":"postman-echo.com","x-amzn-trace-id":"Root=1-5f2ded40-6fd756e8215fc9a2263ddfe0","user-agent":"python-requests/2.21.0","accept-encoding":"gzip, deflate","accept":"*/*","authorization":"Basic cG9zdG1hbjpwYXNzd29yZA=="},"url":"https://postman-echo.com/get?foo1=bar1&foo2=bar2"}
Python POST
API Example
# Here we import the request library
import requests, json
# Here we define the api-endpoint
url = "https://postman-echo.com/post"
# BASIC Authentication
API_USERNAME = 'postman'
API_PASSWORD = 'password'
# Here we define the headers
headers = {'content-type' : 'application/vnd.json+api'}
# Here we enter the json we want ot send to the API
payload = {"foo1":"bar1", "foo2": "bar2"}
# Sending POST request and saving response as response object
r = requests.post(url, headers=headers, auth=(API_USERNAME, API_PASSWORD), json=payload)
# Printing the response object
response = r.text
print("Response:%s"%response)
In your terminal you want to run:
python3 [file_path]
You should see the following output:
Response:{"args":{},"data":{"foo1":"bar1","foo2":"bar2"},"files":{},"form":{},"headers":{"x-forwarded-proto":"https","x-forwarded-port":"443","host":"postman-echo.com","x-amzn-trace-id":"Root=1-5f2dea76-fc0c8a9ca2ef1426d3d96d58","content-length":"32","user-agent":"python-requests/2.21.0","accept-encoding":"gzip, deflate","accept":"*/*","content-type":"application/vnd.json+api","authorization":"Basic cG9zdG1hbjpwYXNzd29yZA=="},"json":{"foo1":"bar1","foo2":"bar2"},"url":"https://postman-echo.com/post"}
Final Thoughts
We covered only GET and POST API calls in this tutorial, but there are additional API calls such as PUT
, PATCH
, and DELETE
. For more information, you can reference Requests documentation. After completing this tutorial, you should be able to build more complicated scripts to submit API requests with Python 3.